Table of Contents
- Introduction
- Why Database Crates Matter
- Diesel: A safe, extensible ORM and Query Builder for Rust
- SQLx: The Rust SQL Toolkit
- Tokio-postgres: A native, asynchronous PostgreSQL client.
- Feature Matrix Comparison
- Making Your Choice
- Conclusion
- References
Introduction
Since its launch in 2015, Rust has established itself as a major player in the programming world, consistently topping charts as one of the most beloved languages among developers. Its promise of performance, robustness, and safety has made it a top choice for everything from system-level software to web development.
One of the pillars in application and web development is the ability to interact seamlessly with databases. Whether you are working on a small-scale utility tool, a web API, or an enterprise-grade application, odds are you'll need to store, retrieve, or manipulate data in a database. That is where Rust's rich ecosystem of libraries, commonly referred to as 'crates', comes into play.
In this article we focus on three crates that standout for an relational SQL database: Diesel
, SQLx
, and Tokio-Postgres
. Each of these offers a distinct set of features, optimizations, and trade-offs, making them popular for different scenarios and requirements.
If you are a Rust developer wondering which database crate to choose for your next project, you've come to the right place. This article will dive into these three top contenders, comparing them on various factors such as:
- Ease of use
- Performance
- Features
- Community support
By the end, you'll have the information you need to make an informed decision for your next Rust project.
Why Database Crates Matter
In today's interconnected, data-driven world, the database is often considered the backbone of any software application. As developers, our interaction with databases is so frequent that the quality of the tools we use for this interaction can significantly impact not just the development process, but also the performance and reliability of the application itself. That is why choosing the database crate is crucial.
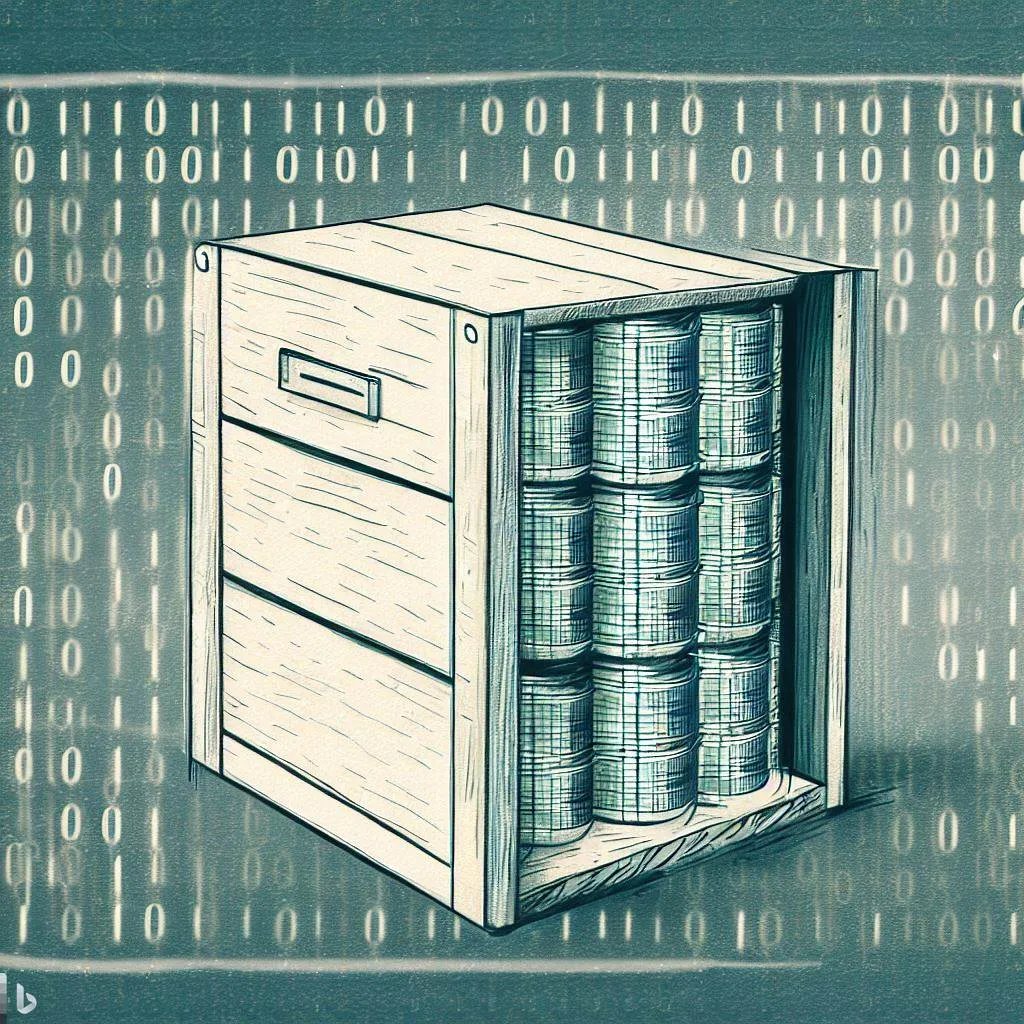
Importance in Application Development
Type Safety
Rust is known for its strong emphasis on type safety and is therefore statically typed, and a great database crate extends this safety. A mistake in a database query can lead to runtime errors or, worse, data corruption. Compile-time SQL verification minimizes these risks, and speed up the feedback cycle.
Performance
Database operations can often be a bottleneck in application performance. A well-optimized database library can make efficient use of resources, enabling your application to run faster and handle more users. This is what we expect from a Rust application.
Developer Productivity
A great database library provides a clean, understandable API that aligns with the language's idioms. This not only makes it easier to write code but also makes it more maintainable.
Scalability
As your application grows, so does the complexity of your database operations. Quality database libraries offer features like connection pooling (i.e. process that maintains a set of open connections to a database, handing them out for repeated use), batch operations, and async support, which are vital for scalability.
Ecosystem Compatibility
The ideal database crate should play well with other libraries and frameworks you may be using. For instance, a web framework like Actix has a great set of code examples, for both Diesel and Tokio-Postgres, making your development process smoother.
Common Challenges in Database Interaction
Connection Management
Managing database connections effectively is a common challenge. Opening too many can stress the database, while too few can lead to underutilization. As developers we need to strike a balance and the crate should assist in this.
Query Complexity
Queries can become increasingly complex. A bad library can make these queries hard to maintain and debug, leading to reduced performance and increased likelihood of errors.
Database Migrations
Managing schema changes or transitioning from one database system to another can be cumbersome. Some database crates offer built-in support for migrations, easing this process.
Asynchronous Programming
(Web) Applications frequently rely on asynchronous I/O operations. While Rust’s async/await syntax is powerful, not all database libraries support it, which can lead to complications in asynchronous applications.
In the sections that follow, we will take a closer look at how Diesel, SQLx, and Tokio-Postgres measure up against these challenges and needs, giving you the insights you require to make the best choice for your project.
Diesel: A safe, extensible ORM and Query Builder for Rust
Diesel is a safe, extensible Object-Relational Mapping (ORM) and query-building library for Rust. The library takes full advantage of Rust's strong typing and ownership model, making it easier for developers to write robust database applications without worrying about SQL injection vulnerabilities or other common pitfalls. As an ORM, Diesel provides abstractions that allow developers to interact with a database in a more idiomatic Rust manner, rather than raw SQL queries.
Key Features
DSL for Query Generation
- Diesel provides a Domain Specific Language (DSL) for creating SQL queries. This DSL allows you to construct queries in a type-safe, Rust-idiomatic way, reducing errors and increasing code readability. Note that the DSL of Diesel is only relevant when working with Diesel. It must be said that the DSL makes it more convenient to write reusable components. Besides the DSL you can always fallback to Raw SQL. Note that it takes some time to get familiarized with DSL.
Strong Type System
- One of Diesel's major highlights is its strong type system that ties into Rust's native type system. This adds an additional layer of safety, ensuring that you are less likely to make mistakes when writing queries.
Asynchronous Support (with diesel-async)
- While Diesel itself is synchronous, you can integrate it with the async runtime by using third-party extensions like
diesel-async
.
- While Diesel itself is synchronous, you can integrate it with the async runtime by using third-party extensions like
Compatibility with Connection Poolers
- While the library itself doesn't offer built-in connection pooling, it is designed to work seamlessly with third-party connection poolers like
bb8
anddeadpool
. This makes it flexible and adaptable to various use-cases.
- While the library itself doesn't offer built-in connection pooling, it is designed to work seamlessly with third-party connection poolers like
Data Migration Tools
- Diesel's robust type system and built-in migration support make it a good fit for data migration tasks.
Community and Ecosystem
Diesel enjoys a robust community and is one of the more mature database libraries in the Rust ecosystem. It has a high number of contributors and maintainers (see Diesel overview), and it is widely used in both open-source and commercial projects. The ecosystem around Diesel is rich, with various plugins and extensions to support features like JSON serialization and integration with other popular Rust libraries.
SQLx: The Rust SQL Toolkit
SQLx is a modern and versatile SQL client for Rust, offering a unique feature set geared towards maintainability and robustness. One of its standout features is the support for compile-time SQL verification. This means that your SQL queries are checked at compile-time for syntax and even some level of semantic correctness, reducing the runtime errors related to database queries.
Key Features
Asynchronous Support
- SQLx is natively designed with asynchronous programming in mind, fully supporting Rust's
async/await
syntax. This makes it an ideal choice for modern web applications that require non-blocking IO.
- SQLx is natively designed with asynchronous programming in mind, fully supporting Rust's
Raw SQL with Compile-time Checks
- Unlike many ORMs that try to abstract SQL away, SQLx embraces it. You write raw SQL queries, which are then verified at compile-time for correctness. This combines the power and flexibility of SQL with the safety guarantees of Rust.
Built-in Connection Pooling
- Connection management is simplified with SQLx's built-in support for database connection pooling called
PgPool
, saving you from having to integrate third-party pooling libraries and optimizing database interactions for you.
- Connection management is simplified with SQLx's built-in support for database connection pooling called
Community and Ecosystem
SQLx has rapidly gained traction in the Rust community, thanks to its modern approach to database interaction. Its GitHub repository is active, with contributions from a variety of developers (see SQLx overview). There is also an active community around it, discussing improvements, best practices, and providing help and support. The ecosystem around SQLx is growing.
Tokio-postgres: A native, asynchronous PostgreSQL client.
Tokio-Postgres is a Rust library designed for working directly with PostgreSQL databases in an asynchronous manner. Unlike Diesel and SQLx, which offer various levels of abstraction and ORM capabilities, Tokio-Postgres is oriented towards a more direct, low-level interaction with the database. This makes it a suitable choice for those who require fine-grained control over their SQL queries and database operations.
Key Features
Asynchronous Support
- Fully integrated with the Tokio runtime, Tokio-Postgres is designed for asynchronous database operations. This enables highly concurrent, non-blocking applications.
Raw SQL Execution
- Tokio-Postgres allows for raw SQL query execution, giving developers maximum control over their database interactions.
Compatibility with Connection Poolers
- While the library itself doesn't offer built-in connection pooling, it is designed to work seamlessly with third-party connection poolers like
bb8
anddeadpool
. This makes it flexible and adaptable to various use-cases.
- While the library itself doesn't offer built-in connection pooling, it is designed to work seamlessly with third-party connection poolers like
Community and Ecosystem
Tokio-Postgres benefits from being a part of the larger Tokio ecosystem, which is widely adopted for asynchronous programming in Rust. The library has a somewhat specialized use-case, but it is well-maintained and has a solid community of users and contributors. Although not as extensive as the ecosystems around Diesel or SQLx, there is community support for extending its functionalities through third-party libraries and poolers.
Feature Matrix Comparison
Feature | diesel | sqlx | tokio-postgres |
---|---|---|---|
Asynchronous support | Yes with diesel-async | Yes | Yes |
Synchronous support | Yes | No | Yes (postgres ) |
ORM (Object Relational Mapping) | Yes | No | No |
Compile-time SQL verification | Yes | Yes | No |
Raw SQL execution | Yes | Yes | Yes |
Connection pooling | Via bb8 or deadpool | Built-in | Via bb8 or deadpool |
Macros for query generation | Yes | Yes | No |
Supports multiple databases | Yes | Yes | No (PostgreSQL specific) |
Integrated migration tools | Yes | Yes | No |
Query Interface | DSL & Raw SQL | Raw SQL with Macros | Raw SQL |
In this context:
- DSL (Domain Specific Language):
diesel
offers a Rust-based DSL to construct queries, which allows for more type safety and is more idiomatic to Rust. You can also use raw SQL if desired. - Raw SQL with Macros:
sqlx
primarily uses raw SQL but provides macros to enable compile-time verification. - Raw SQL:
tokio-postgres
andpostgres
are more focused on raw SQL without much abstraction.
It is worth noting that while the above mentions the primary query interfaces for each crate, many of these libraries offer a blend of features, and developers can often choose between raw SQL or more abstracted query generation based on their needs.
Making Your Choice
After diving into each of these prominent Rust database crates, the next step is to decide which one aligns most closely with your project’s specific needs. While all three libraries offer a robust set of features, the best choice will depend on a variety of factors, such as performance requirements, type safety, level of control, and the kind of community support you're looking for.
Performance
If your primary concern is high-performance, non-blocking IO, you might lean towards SQLx or Tokio-Postgres, both of which are designed for asynchronous operations. Diesel, while robust and feature-rich, operates synchronously by default, although there are third-party extensions for async support.
Type Safety
When it comes to type safety, Diesel's strong integration with Rust's type system offers arguably the most robust compile-time checks. SQLx also provides compile-time SQL verification, making it a good choice for projects where type safety is a priority.
Level of Control
If you need fine-grained control over your SQL queries and prefer to work at a lower level, Tokio-Postgres might be the way to go. Diesel and SQLx provide various levels of abstraction and ORM capabilities, which could be seen as either an advantage or a limitation, depending on your project's needs.
Community Support
Community engagement is another crucial factor. A well-supported library often translates into better documentation, more third-party extensions, and quicker help when you run into issues. Diesel and SQLx have larger communities and more active repositories compared to Tokio-Postgres. However, Tokio-Postgres benefits from being part of the larger Tokio ecosystem, which is itself widely adopted.
Project Maturity
Finally, consider the maturity of your selected library. More established projects like Diesel have been battle-tested in a variety of environments, which may give you more confidence in their stability. SQLx, while newer, has rapidly gained maturity and traction. Tokio-Postgres, being more specialized, might not have the same breadth of usage but is stable and reliable for its intended use-cases.
Conclusion
Choosing a database library is often a trade-off between various factors such as performance, type safety, level of abstraction, and community support. Your project's specific needs will guide your choice, and fortunately, whether you choose Diesel for its powerful ORM capabilities, SQLx for its asynchronous support and type safety, or Tokio-Postgres for low-level control, you're in good hands.
My Two Cents
My go-to choice has to be SQLx, and for good reason. Not only does it offer native support for asynchronous programming, but it also comes with built-in connection pooling, elevating its efficiency to the next level. Moreover SQLx has compile-time SQL verification, ensuring that your queries are bulletproof before they even hit the runtime. To top it all off, it seamlessly integrates with the Actix Web framework, making it an unparalleled tool in my development arsenal.
References
Diesel overview
- Snapshot Date: September 8, 2023
- GitHub Repository: Diesel GitHub
- Stars: 10900+
- Contributors: 308
- Documentation: Diesel Documentation
SQLx overview
- Snapshot Date: September 8, 2023
- GitHub Repository: SQLx GitHub
- Stars: 9700+
- Contributors: 322
- Documentation: SQLx Documentation
Tokio-postgres overview
- Snapshot Date: September 8, 2023
- GitHub Repository: Tokio-postgres GitHub
- Stars: 3000+
- Contributors: 120
- Documentation: Tokio-postgres Documentation