We all make errors. But how to handle them?
I am excited to bring you a new edition of our newsletter, focused on a topic that is crucial to every programmer: error handling in Rust. No matter how experienced or talented you are, errors are an inevitable part of the programming process. But do not worry, Rust provides a robust and efficient way to handle them.
In this edition, we will dive into the world of error handling in Rust and explore how you can handle errors in your programs with ease. From the Result type and the ? operator, to the match operator and beyond, we will cover all the essential elements of error handling in Rust. So, without further ado, let’s dive in!
Introduction to Error Handling
Rust achieves strict handling of errors by using its type system to force it at compile time. In other words, the code will not compile and therefore not run. Which makes writing incorrect code harder from the start.
Errors in Rust can be classified into two categories:
- Recoverable errors
- Unrecoverable errors
Recoverable errors are errors that can be handled and recovered from within the program. These errors are usually indicated by the Result type, which represents the result of an operation that might fail.
Unrecoverable errors, on the other hand, are errors that cannot be handled within the program and cause the program to terminate immediately. These errors are usually indicated by using the panic! macro or assert macro that calls panic!, which stops the program and displays an error message. Unrecoverable errors are typically used for cases where something unexpected has occurred, such as an assertion failure or a bug in the code.
In my opinion, there is no justification for intentionally causing a panic. This is because panics can always be replaced with recoverable errors, which provide a more flexible and controlled method of handling errors. So we will focus on the recoverable errors.
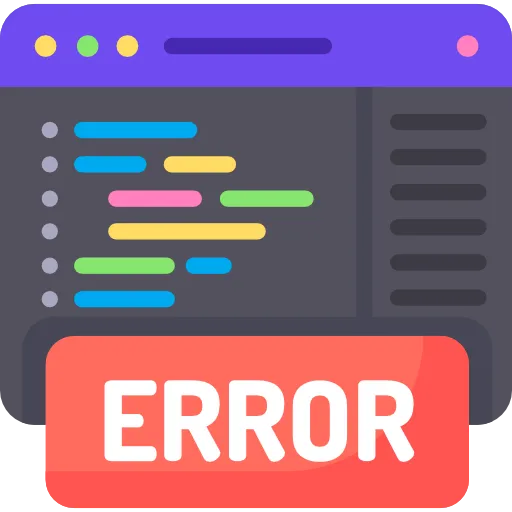
Recoverable errors
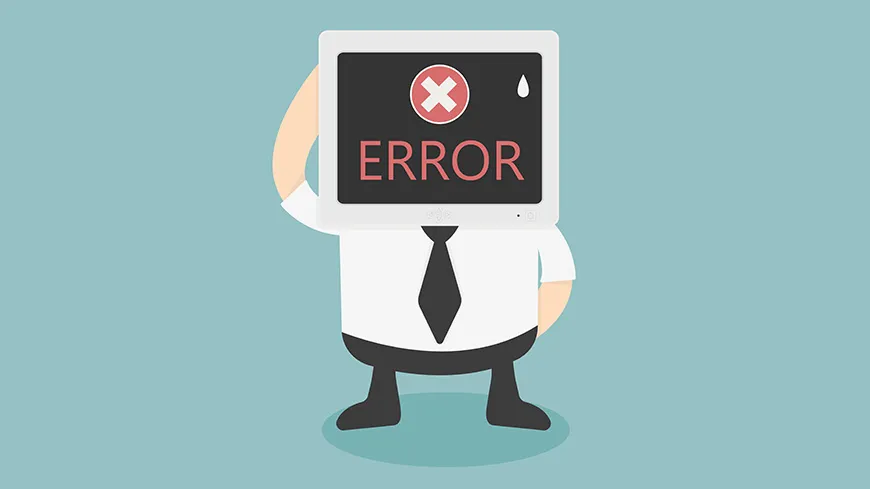
When an operation returns Ok(T), it means that the operation was successful and the value of type T is returned.
When an operation returns Err(E), it means that the operation failed and an error of type E is returned.
The programmer can then handle the error by using techniques such as pattern matching, propagating the error up the call stack with the ? operator, or by using error-handling functions such as unwrap() or expect().
Besides the Result
type there is another important type called Option
. In Rust, the Option
type is a way to represent the presence or absence of a value. It is a generic enum with two variants: Some(T) and None. Some(T) holds a value of type T, while None represents the absence of a value.
For example, you can use an Option
value to represent the result of a function that may or may not find a value, like searching a vector for an item. Instead of returning an error code or throwing an exception, the function would return Some(T) if a value was found and None otherwise.
In this way, the Option
type can be seen as part of error handling because it provides a way to handle the absence of a value in a type-safe and explicit way, without relying on error codes or exceptions.
The following links might be useful to you to dive deeper into the discussed topics:
- The Rust Book: Recoverable Errors
- Error struct in the standard library
- The
Result
type in the standard library - Great blog post by Burntsushi (Andrew Gallant)
- Another blog post on unwrap by Burntsushi
Snippets
- Rust as a programming language for automotive software?
- Did you know there is also a Rust Trends LinkedIn Page?
- Rust 1.67.1, February the 9th. Do not forget to run
rustup update
Enjoy your Sunday, and have a great week ahead.
Thanks for reading!
Bob Peters
Feel free to connect with me on LinkedIn